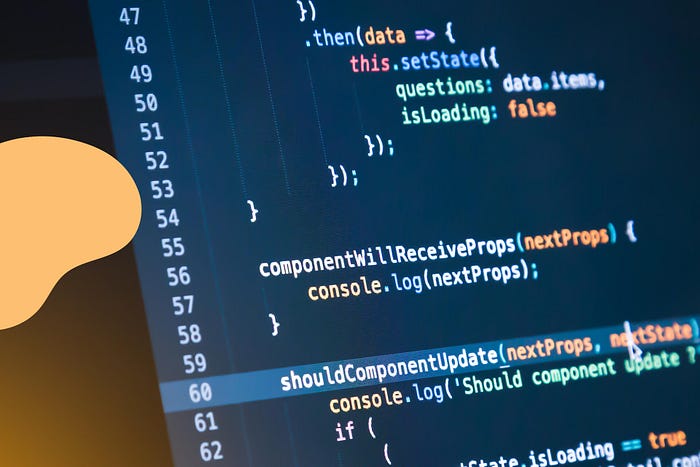
React has become the go-to library for building user interfaces, whether it’s for web applications, mobile apps with React Native, or even server-side rendering with React Server Components. However, the journey to becoming a proficient React developer involves more than just writing components. This comprehensive guide will explore best practices, code structure, performance optimization, accessibility, security considerations, the React ecosystem, and real-world applications.
Table of Contents
React Best Practices
Code Structure and Organization
Optimizing Performance
Accessibility in React
Security Considerations
React Ecosystem
React Native
React Server Components
React Tools and DevOps
Popular React Libraries
React Best Practices
Code Structure and Organization
Efficient code organization is crucial for maintaining a React project. A well-structured codebase enhances collaboration and ensures scalability. Here are some best practices:
Folder Structure
Keep your project organized with a clear folder structure. One popular approach is to group files by feature or domain, for example:
/src
/components
/Button
Button.js
Button.test.js
/User
UserCard.js
UserList.js
/containers
/Home
Home.js
Home.test.js
/utils
api.js
/styles
main.css
/context
authContext.js
/routes
AppRouter.js
/constants
routes.js
Component Organization
Divide components into functional, stateful, and presentational components. This separation of concerns makes your code more maintainable and reusable.
// Functional Component
function Header() {
return <header>My App</header>;
}
// Stateful Component
class UserProfile extends React.Component {
constructor(props) {
super(props);
this.state = {
user: null,
};
}
// ...
}
// Presentational Component
function UserInfo({ name, email }) {
return (
<div>
<h2>{name}</h2>
<p>{email}</p>
</div>
);
}
Container and Presentational Components
Use a container/presentational component pattern. Containers manage data and state, while presentational components focus on rendering the UI.
// Container Component
class UserContainer extends React.Component {
// ...
render() {
return (
<UserList users={this.state.users} />
);
}
}
// Presentational Component
function UserList({ users }) {
return (
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
}
Optimizing Performance
React’s virtual DOM provides excellent performance out of the box, but you can take additional steps to optimize your application further.
Memoization
Use the React.memo
higher-order component to memoize functional components. This prevents unnecessary renders.
const MemoizedComponent = React.memo(function MyComponent(props) {
// ...
});
ShouldComponentUpdate
In class components, implement shouldComponentUpdate
to control rendering. This allows you to optimize when and how your component updates.
class MyComponent extends React.Component {
shouldComponentUpdate(nextProps, nextState) {
// Check if rendering is necessary
return this.props.data !== nextProps.data;
}
// ...
}
Lazy Loadin
Load components lazily using React’s lazy
and Suspense
. This reduces the initial bundle size and improves load times.
const MyLazyComponent = React.lazy(() => import('./MyLazyComponent'));
function MyParentComponent() {
return (
<React.Suspense fallback={<div>Loading...</div>}>
<MyLazyComponent />
</React.Suspense>
);
}
Code Splitting
Break your code into smaller chunks to take advantage of code splitting. Tools like Webpack can help achieve this.
import { add } from './math';
const result = add(2, 3);
Accessibility in React
Creating accessible applications is not only important for inclusivity but often a legal requirement. React provides tools to make your app accessible.
Semantic HTML
Use semantic HTML elements to create a meaningful structure for your components. Avoid using non-semantic elements for important content.
// Bad
<div onClick={handleClick}>Click me</div>
// Good
<button onClick={handleClick}>Click me</button>
ARIA Roles and Labels
When semantic HTML isn’t sufficient, use ARIA roles and labels to provide accessibility information.
<div role="button" tabIndex="0" onClick={handleClick} aria-label="Click me">
Click me
</div>
Focus Management
Ensure proper focus management by using the autoFocus
attribute and JavaScript methods like focus()
and blur()
.
class Modal extends React.Component {
componentDidMount() {
this.modalRef.focus();
}
render() {
return <div ref={node => (this.modalRef = node)} tabIndex={-1}>Modal content</div>;
}
}
Security Considerations
React applications are susceptible to various security threats. Here are some best practices to enhance your app’s security.
Avoid Cross-Site Scripting (XSS)
Sanitize user input and use React’s built-in mechanisms, such as {dangerouslySetInnerHTML}
, with caution. Always validate and sanitize user-generated content.
const userInput = '<script>alert("XSS")</script>';
const sanitizedInput = sanitize(userInput);
<div dangerouslySetInnerHTML={{ __html: sanitizedInput }}></div>
Prevent Cross-Site Request Forgery (CSRF)
Use anti-CSRF tokens to protect against CSRF attacks. Ensure that all state-changing requests (e.g., POST, PUT) require a valid token.
const token = getCSRFToken();
fetch('/api/update', {
method: 'POST',
body: JSON.stringify({ data: 'newData', _csrf: token }),
headers: {
'Content-Type': 'application/json',
},
});
Content Security Policy (CSP)
Implement a Content Security Policy to restrict the sources from which resources can be loaded. This helps mitigate the risk of code injection.
<meta http-equiv="Content-Security-Policy" content="default-src 'self'">
React Ecosystem
React has a vast ecosystem with tools and libraries that can enhance your development experience.
React Native
If you want to build mobile applications, React Native is the way to go. It allows you to write code in React and deploy it to both iOS and Android.
import React from 'react';
import { Text, View } from 'react-native';
function App() {
return (
<View>
<Text>Hello, React Native!</Text>
</View>
);
}
React Server Components
React Server Components are a cutting-edge technology that enables server-side rendering with the flexibility of React. It allows you to build highly interactive web applications with server-rendered components.
import { ssr } from 'react-server-components';
export default ssr`
<MyComponent />
`;
React Tools and DevOps
Leverage tools and DevOps practices to streamline your React development workflow. Popular tools include:
- Webpack: Bundles and optimizes your code.
- Babel: Transpiles modern JavaScript for compatibility.
- ESLint: Enforces coding standards and identifies issues.
- Jest: Testing framework for React applications.
- Cypress: End-to-end testing for web applications.
- Storybook: Helps you develop, document, and test React components in isolation.
Popular React Libraries
Explore various React libraries that can save you time and effort in development:
- Redux: A predictable state container for managing application state.
- React Router: Routing library for navigation in React applications.
- Axios: A popular HTTP client for making network requests.
- Styled-components: Library for styling React components with CSS-in-JS.
- Formik: A library for building forms in React applications.
Conclusion
In conclusion, mastering React involves adhering to best practices in code structure, optimizing performance, ensuring accessibility, and addressing security concerns. Expanding your knowledge of the React ecosystem, including React Native and React Server Components, can unlock new avenues for application development. Utilizing popular libraries and tools streamlines your workflow, continuous learning and application of these practices will help you excel in this ever-evolving field. Happy coding!
To be continued…